What is the FileMaker Data API?
If you use PHP to access FileMaker data, you’re in the right place.
Starting with FileMaker version 17, FileMaker Server offers REST access to your FileMaker databases and has been gradually deprecating native PHP functionality.
If you use REST as part of your PHP solution, you would usually need to write a series of cURL functions and you would have to manually manage connecting & disconnecting from your server, and saving your tokens.
This is a pain!
We know it’s a pain, because we cut our teeth rolling REST functionality into soSIMPLE Calendar.
FREE fmREST.php
Simplifies & manages PHP connections to FileMaker’s REST-based Data API.
We created this class file to make it easier to manage dynamic REST sessions for soSIMPLE server and web products, and for our custom development. The goal of the class file was to help PHP developers start using the new REST engine as quickly and easily as possible.
We’ll also be updating it with new features. If you’d like to add something to it, please let us know.
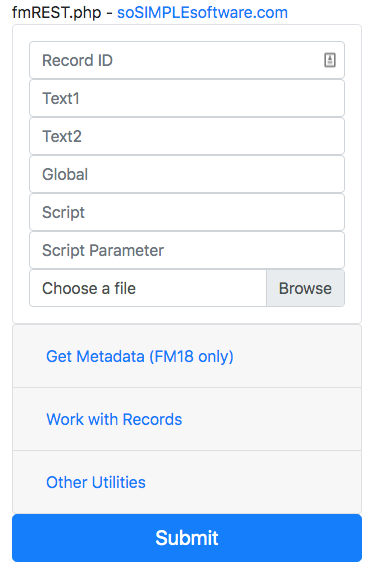
What fmREST.php does:
- Makes every REST call available as a PHP function.
- Automatically login into FileMaker Server whenever you call any REST functions
- Saves your token for 15 minutes to reuse
- Checks for a broken or disconnected token and automatically reconnects and runs your function again
- Includes a sample file illustrating all the functions
Where do I get it?
include_once (‘fmREST.php’);
$fm = new fmREST ($host, $db, $user, $pass [, $layout]);
$fm = new fmREST ();
$fm -> host = ‘myhost.com’;
$fm -> db = ‘my database.fmp12’;
$fm -> user = ‘admin’;
$fm -> pass = ‘mySecretPassword’;
Note: layout is now optional when setting up your connection. If you set it here, it will be used as a default for each function, or you can include the layout when calling the function.
3. Call your functions:
fmREST will automatically log in for you as necessary, as each function is called.
Important note: since we use a cookie to keep track of your token, your first function, or a specific call to the login function should be called before any visible content on the page.
How the functions work:
The functions that are part of this class use the same name and the same construct as the Data API. The only substantial difference is that you can pass the parameters as either JSON strings, or as PHP arrays. We find that native PHP arrays have been the easiest method.
For specific reference of the expected parameters, and results refer to the API documentation, located on your FileMaker Server. The documentation is located:
https:///fmi/data/apidoc/. There’s also a wealth of information here:
For FileMaker 17: https://fmhelp.filemaker.com/docs/17/en/dataapi/
For FileMaker 18: https://fmhelp.filemaker.com/docs/18/en/dataapi/
For FileMaker 19+: https://help.claris.com/en/data-api-guide/
Function Reference:
WORKING WITH EARLIER VERSIONS OF FILEMAKER: The class file is set to work with FileMaker 19 by default. To work with earlier versions, change the following two settings, right after $fm=new fmREST() call:$fm -> version = “v1”;
$fm -> fmversion = 17;
$result = $fm -> login ();
- We use the user name and password from when you instantiated the class. This is the only function that doesn’t take parameters the same way as the API Doc.
- You don’t need to call this explicitly. It will be called automatically whenever any data function is called.
- You don’t Whether explicitly called, or part of another function, the login should happen before any content is shown on the page. This allows the class file to set a cookie, which in turn allows successive call to use the same token.to call this explicitly. It will be called automatically whenever any data function is called.
$result = $fm -> logout ([token]);
- The token parameter is optional. fmREST will use the token stored in your cookie if you don’t provide one.
- We don’t log out automatically when functions are called. Doing so would add to the overhead of every call, defeating the purpose of managing tokens.
- FileMaker Server will automatically disconnect you after 15 minutes, or you can call this function to manually disconnect the session.
$result = $fm -> validateSession ([token]);
- The token parameter is optional. fmREST will use the token stored in your cookie if you don’t provide one.
- messages[0][code] returns a error zero ( 0 ) for a valid token, and error 952 for an invalid token (see API Doc for more info)
EXAMPLE:
$record[‘my_field_name’] = ‘my_field_value’;
$record[‘another field’] = ‘another value’;
$data[‘fieldData’] = $record;
$data[‘script’] = ‘a script I want to run’;
$data[‘script.param.’] = ‘a parameter to pass for the script above’;
$result = $fm -> createRecord ($data);
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- please note how the record data is an array of fields, wrapped within another array
- all field data needs to be wrapped in quotation marks – even number fields
- scripts can be set to run during specific parts of each routine. See API docs for more details.
FORMAT: $result = $fm -> deleteRecord ($recordId [, $layout]);
EXAMPLE:
$recordId = 1234;
$result = $fm -> deleteRecord ($recordId);
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- $recordId is the ID of the record – not the primary key field. You can find it in FileMaker Pro by calling get(recordid). It’s also part of every record returned from the API as $result [‘recordId’];
- scripts can be set to run during specific parts of each routine. See API docs for more details.
FORMAT: $result = $fm -> editRecord ($recordId, $data [, $layout]);
RESULT:
$recordId = 1234;
$record[‘my_field_name’] = ‘my_field_value’;
$record[‘another field’] = ‘another value’;
$data[‘fieldData’] = $record;
$result = $fm -> editRecord ($recordId, $data);
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- please note how the record data is an array of fields, wrapped within another array
- all field data needs to be wrapped in quotation marks – even number fields
- $recordId is the ID of the record – not the primary key field. You can find it in FileMaker Pro by calling get(recordid). It’s also part of every record returned from the API as $result [‘recordId’];
- scripts can be set to run during specific parts of each routine. See API docs for more details.
FORMAT: $result = $fm -> getRecord ($recordId [, $parameters, $layout]);
EXAMPLE:
$recordId = 1234;
$parameters [‘script’] = ‘my script name’;
$parameters [‘script.param’] = ‘a parameter for that script’;
$result = $fm -> getRecord ($recordId, $parameters);
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- $recordId is the ID of the record – not the primary key field. You can find it in FileMaker Pro by calling get(recordid). It’s also part of every record returned from the API as $result [‘recordId’];
- scripts can be set to run during specific parts of each routine and other parameters can specify portal data returned and more. See API docs for more details.
FORMAT: $result = $fm -> getRecords ($parameters [,$layout]);
EXAMPLE:
$parameters [‘_offset’] = 1;
$parameters [‘_limit’] = 50;
$parameters [‘script’] = ‘my script name’;
$parameters [‘script.param’] = ‘a parameter for that script’;
$result = $fm -> getRecords ($parameters);
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- scripts can be set to run during specific parts of each routine and other parameters can specify portal data returned and more. See API docs for more details.
- please note the preceding underscore in some of the parameters (“_limit” for example) on this call, as specified in the API docs.
FORMAT: $result = $fm -> findRecords ($data [, $layout]);
EXAMPLE:
$request1[‘my_field_name’] = ‘my_field_value’;
$request1[‘another field’] = ‘another value’;
$request2[‘my_field_name’] = ‘my_field_value’;
$query = array ($request1, $request2);
$data[‘query’] = $query;
$data[‘limit’] = 2;
$data[‘script’] = ‘find script’;
$data[‘script.param’] = ‘script parameter’;
$result = $fm -> findRecords ($data);
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- you can have any number of requests be part of a query by adding elements to the $query variable.
- you can also add sort, offset, range and portal by adding these parameters to the $data variable.
- please note how the request data is an array of fields, which is wrapped within another array (for each request), then wrapped into another array before it’s sent to the findRecords function.
- all field data needs to be wrapped in quotation marks – even number fields
- scripts can be set to run during specific parts of each routine. See API docs for more details.
FORMAT: $result = $fm -> uploadContainer ($recordId, $fieldName, $file [, $layout] );
EXAMPLE:
$recordId = 1234;
$fieldName = ‘container’;
$file = $_FILES[‘file’];
$result = $fm -> uploadContainer ($recordId, $fieldName, $file );
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- $recordId is the ID of the record – not the primary key field. You can find it in FileMaker Pro by calling get(recordid). It’s also part of every record returned from the API as $result [‘recordId’]
FORMAT: $result = $fm -> setGlobalFields ($data);
EXAMPLE:
$globals[‘my toc::my field’] = ‘my_field_value’;
$globals[‘another toc::another field’] = ‘another value’;
$data[‘globalFields’] = $globals;
$result = $fm -> setGlobalFields ($data);
- please note how the field data is an array of fields, wrapped within another array
- all field data needs to be wrapped in quotation marks – even number fields
- the field name needs to be an absolute reference, including the table occurrence name
FORMAT: $result = $fm -> executeScript ($scriptname [,$scriptparameter, $layout]);
EXAMPLE:
$result = $fm -> executeScript ($_REQUEST[‘Script’],$_REQUEST[‘Parameter’]);
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- New for FileMaker 18
$result = $fm -> productInfo ();
$result = $fm -> databaseNames ();
$result = $fm -> layoutNames ();
$result = $fm -> scriptNames ();
$result = $fm -> layoutMetadata ([$layout]);
$result = $fm -> oldLayoutMetadata ([$layout]);
- layout is optional if you’ve included a layout when you called “new fmREST()” function.
- New for FileMaker 18
DEBUGGING:
You now have three options for showing the debug output of fmREST.php:
- $fm -> show_debug = true; – turn on debugging when instantiating class – will automatically show the debug output after your code has executed.
- $fm -> show_debug = “HTML”; – turn on debugging when instantiating class – will automatically show the debug output in HTML format instead of as an array.
- print_r ($fm -> debug_array); – manually output the contents of the debug array wherever you want it on your page, or use it elsewhere.
$fm -> version = “v1”;
$fm -> fmversion = 17;